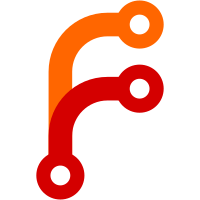
`gen` is a reserved keyword in rust 2024, making it very awkward to use as a module/variable name.
54 lines
1.4 KiB
Rust
54 lines
1.4 KiB
Rust
mod util;
|
|
use schemars::JsonSchema;
|
|
use util::*;
|
|
|
|
fn schema_fn(generator: &mut schemars::SchemaGenerator) -> schemars::Schema {
|
|
<bool>::json_schema(generator)
|
|
}
|
|
|
|
struct DoesntImplementJsonSchema;
|
|
|
|
#[allow(dead_code)]
|
|
#[derive(JsonSchema)]
|
|
struct Struct {
|
|
#[schemars(schema_with = "schema_fn")]
|
|
foo: DoesntImplementJsonSchema,
|
|
bar: i32,
|
|
#[schemars(schema_with = "schema_fn")]
|
|
baz: DoesntImplementJsonSchema,
|
|
}
|
|
|
|
#[test]
|
|
fn struct_normal() -> TestResult {
|
|
test_default_generated_schema::<Struct>("schema_with-struct")
|
|
}
|
|
|
|
#[allow(dead_code)]
|
|
#[derive(JsonSchema)]
|
|
pub struct Tuple(
|
|
#[schemars(schema_with = "schema_fn")] DoesntImplementJsonSchema,
|
|
i32,
|
|
#[schemars(schema_with = "schema_fn")] DoesntImplementJsonSchema,
|
|
);
|
|
|
|
#[test]
|
|
fn struct_tuple() -> TestResult {
|
|
test_default_generated_schema::<Tuple>("schema_with-tuple")
|
|
}
|
|
|
|
#[derive(JsonSchema)]
|
|
pub struct Newtype(#[schemars(schema_with = "schema_fn")] DoesntImplementJsonSchema);
|
|
|
|
#[test]
|
|
fn struct_newtype() -> TestResult {
|
|
test_default_generated_schema::<Newtype>("schema_with-newtype")
|
|
}
|
|
|
|
#[derive(JsonSchema)]
|
|
#[schemars(transparent)]
|
|
pub struct TransparentNewtype(#[schemars(schema_with = "schema_fn")] DoesntImplementJsonSchema);
|
|
|
|
#[test]
|
|
fn struct_transparent_newtype() -> TestResult {
|
|
test_default_generated_schema::<TransparentNewtype>("schema_with-transparent-newtype")
|
|
}
|